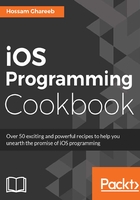
Using Enums with raw values
We saw how easy it is to create enums with raw values. Now, let's take a look at how to get the raw value of enums or create enums back using raw values.
We already saw how to get the raw value from enum by just calling .rawValue to return the raw value of the enum case.
To initialize an enum with a raw value, the enum should be declared with a type; so in that case, the enum will have a default initializer to initialize it with a raw value. An example of an initializer will be like this:
let httpCode = HTTPCode(rawValue: 404) // NotFound let httpCode2 = HTTPCode(rawValue: 1000) // nil
The rawValue initializer always returns an optional value because there will not be any matching enum for all possible values given in rawValue. For example, in case of 404, we already have an enum whose value is 404. However, for 1000, there is no enum with such value, and the initializer will return nil in that case. So, before using any enum initialized by the rawValue initializer, you have to check first whether the value is not equal to nil; the best way to check for enums after initialization is by this method:
if let httpCode = HTTPCode(rawValue: 404){ print(httpCode) } if let httpCode2 = HTTPCode(rawValue: 1000){ print(httpCode2) }
The condition will be true only if the initializer succeeds to find an enum with the given rawValue.