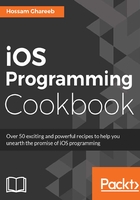
How it works...
Now, you have a new type in your program called Monster, which takes one value of given four values. The values are defined with the case keyword followed by the value name. You have two options to list your cases; you can list each one of them in a separate line preceded by the case keyword, or you can list them in one line with a comma separation. I prefer using the first method, that is, listing them in separate lines, as we will see later that we can add raw values for cases that will be more clear while using this method.
If you come from a C or Objective-C background, you know that the enums values are mapped to integer values. In Swift, it's totally different, and they aren't explicitly equal to integer values.
The first variable monster1 is created using the enum name followed by '.' and then the type that you want. Once monster1 is initialized, its type is inferred with Monster; so, later you can see that when we changed its value to Bear, we have just used the '.' operator as the compiler already knows the type of monster1. However, this is not the only way that you will use enums. Since enums is a group of related values, so certainly you will use it with control flow to perform specific logic based on its value. The switch statement is your best friend in that case as we saw in the monsterPowerFromType() function.
We've created a function that returns the monster power based on its type. The switch statement checks all values of monster with '.' followed by an enum value. As you already know, the switch statement is exhaustive in Swift and should cover all possible values; of course, you can use default in case it's not possible to cover all, as we saw in the canMonsterSwim() function. The default statement captures all non-addressed cases.