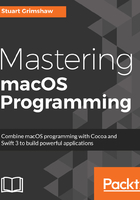
switch
Swift's switch statement (try saying that three times in quick succession) is a lot more flexible than Objective C's, and indeed most other languages'. We'll look briefly at the basics here, leaving the more advanced stuff for Chapter 5, Advanced Swift.
Firstly, switch will test any kind of value; it doesn't need to be an Int. We might have a String value called color:
switch color
{
case "red":
print("The color is red")
case "yellow":
print("The color is yellow")
case "green":
print("The color is green")
default:
print("You are not at a traffic light")
}
Also, a case can be quite flexible. Let's assume we have an integer, luckyNumber:
switch luckyNumber
{
case 0:
print()
case 1,2:
print()
case 3..<10:
print()
case 10...20:
print()
default:
print()
}
As you can see here, we can test against several cases at once, as in case1,2:
We can also test whether x falls within the range 3..<10 (which does not include 10) or 10...20 (which does include 20; note the dots).
- 0..<10 produces the range 0 to 9
- 0...10 produces the range 0 to 10
Finally, the default case covers all other integers.
A switch block must be exhaustive, so we must add the default case to cover all the integers we haven't dealt with explicitly. Xcode will remind us if we don't.
If the value to be tested is a Bool or an Enum (see Enums in this chapter), then we will possibly provide a case for each possible value, and will not, therefore, need a default case.
You may have noticed there are no break statements. That's because the behavior of switch in Swift has no implicit fall-through; once a match has been found, no further cases are tested.