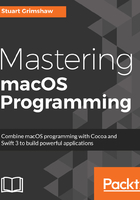
Unwrapping an optional
When we need to test an optional value for nil, we can do so with a simple if statement:
if squareOfTwo != nil
{
//
}
Within the braces of the preceding code, we might need to use the value of squareOfTwo, which we can forceunwrap as follows:
if squareOfTwo != nil
{
var x = squareOfTwo!
}
Note the exclamation mark.
We also can test the optional and assign its value to a constant with an if let statement, as follows:
if let answer = squareOfTwo
{
var x: Int
x = answer
}
The constant answer is not an optional; it has been unwrapped, and so its value can be assigned to x, which is not an optional.
There is nothing to stop us assigning an unwrapped optional to a constant or variable with the same name:
if let squareOfTwo = squareOfTwo
{
var x: Int
x = squareOfTwo
}
After the if let statement, the new squareOfTwo is not an optional. However, this way of unwrapping may make your code a little confusing, since the squareOfTwo inside the braces and the squareOfTwo outside are different types! But this is a common pattern, and it has its uses.
We will learn more about optionals when we get to Chapter 5, Advanced Swift. In the meantime, here are the main points to remember about optionals:
- Optional and non-optional versions of a data type are not the same data type
- Always use optional data types where a variable's value can be nil
- Don't force unwrap an optional unless you're sure it cannot be nil
Using an optional will let Swift save you from any number of programming errors and needlessly risky programming practices. They do take a little getting used to, to be sure, but once you have been using them for a while, you are likely to value them as highly as most developers using Swift do.