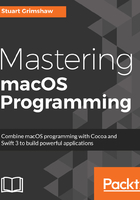
KVO - compliant classes
Let's first create a class that will satisfy the very simple demands of KVO.
Create a new Xcode project, and name it PeopleWatcher:
- Using command + N to create a new file, select Swift File and name it Person.swift.
- Change the import statement in the file to this:
import AppKit
This will allow us to use the classes of AppKit.
- Below the import statement, add the following code:
class Person: NSObject
{
dynamic var name: String
dynamic var busy: Bool
dynamic var shirtColor: NSColor
init(name: String,
busy:Bool,
shirtColor: NSColor)
{
self.name = name
self.busy = busy
self.shirtColor = shirtColor
super.init()
}
}
We can see that the Person class has three properties: name, which is a String object; busy , which is a Bool; and shirtColor, which is an NSColor, which is a class from AppKit. So here we have properties both from Swift and AppKit.
As one would expect, Person objects will be ranked according to the color of their shoes.
So what's with the dynamic keyword? Well, this asks the Swift compiler to use something called dynamic dispatch, which in turn allows something called interception, which is part of the Objective C messaging system, and is basically something that falls outside of the scope of this book except to say:
And the rest we'll leave for another day.
The Person class has an init(name: busy: shirtColor:) method, and notice there's no override here, since NSObject, the superclass, has an init() method, but no init(name: busy: shirtColor:)method. Person is a class, not a struct, so we don't get a member--wise initializer for free.
So that's done. With the addition of dynamic to the properties, the class becomes KVO--compliant. How easy was that?