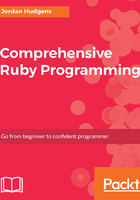
Method argument code examples
I'm going to start with a very basic example that prints out a user's full name. This is actually a code snippet I took from a real-world code project:
def full_name(first_name, last_name)
first_name + " " + last_name
end
puts full_name("Jordan", "Hudgens")
As you'll see, this prints out my full name.

The syntax for how to pass arguments to a method is to simply list them off on the same line that you declare the method. From here, you can treat the names that you supplied as arguments (in this case, first_name and last_name) as variables in the method.
Now there is also an alternative syntax that we can use that's quite popular among Ruby developers for passing arguments to methods. Here is a slightly updated version of the code example from earlier:
def full_name first_name, last_name
first_name + " " + last_name
end
puts full_name"Jordan", "Hudgens"
Notice how all of the parentheses have been taken away? There is some debate on which option is better. I personally prefer to write less code when I have the chance to, so I prefer the second syntax. However both options will work well.