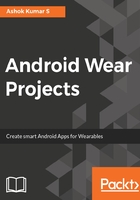
Building the User Interface
The User Interface (UI) needs to be simple and glanceable. For managing different form factors of a Wear device, such as a circle and square, we will be using a container, which is also referred to as a screen shape-aware frame layout. For getting the best listing experience, we will be using the latest component, WearableRecyclerView, from the Wear 2.0 support library. WearableRecyclerView has the best architecture, which is identical to RecyclerView.
For activity_main.xml, add the following code:
<?xml version="1.0" encoding="utf-8"?> <android.support.wearable.view.BoxInsetLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/container" tools:context="com.ashok.packt.wear_note_1.activity.MainActivity" tools:deviceIds="wear" app:layout_box="all" android:padding="5dp"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:layout_gravity="center"> <EditText android:layout_width="match_parent" android:layout_height="50dp" android:id="@+id/edit_text" android:gravity="center" android:inputType="textCapSentences|textAutoCorrect" android:layout_gravity="center" android:imeOptions="actionSend" android:textColorHint="@color/orange" android:hint="@string/add_a_note"/> <android.support.wearable.view.WearableRecyclerView android:id="@+id/wearable_recycler_view" android:layout_width="match_parent" android:layout_height="match_parent"/> </LinearLayout> </android.support.wearable.view.BoxInsetLayout>
Before adding all the UI components, let's add all the string resources to be used. This resource is assumed for the operation that we have planned to implement in the Wear note app.
Add the following resources in strings.xml under the res directory and the value directory:
<resources> <string name="app_name">Wear-note-1</string> <string name="hello_world">Hello World!</string> <string name="add_a_note">Add a Note</string> <string name="delete">Remove</string> <string name="note_saved">Saved</string> <string name="note_removed">Note Removed</string> <string name="cancel">Cancelled</string> </resources>
Next, let's add the WearableRecyclerView list item layout. It's called each_item.xml. For creating a new layout, go to the res directory and navigate to the layout directory. Right-click and go to New and then select the Layout resource file. Once the layout file is created, add the following code:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:layout_gravity="center" android:clickable="true" android:background="?android:attr/selectableItemBackground" android:orientation="vertical"> <TextView android:id="@+id/note" android:layout_width="wrap_content" android:layout_height="wrap_content" android:gravity="center" android:layout_gravity="center" tools:text="note"/> <View android:layout_width="match_parent" android:layout_height="1px" android:layout_marginLeft="5dp" android:layout_marginRight="5dp" android:background="@android:color/darker_gray"/> </LinearLayout>