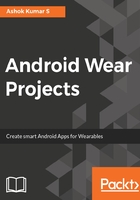
Let's get started by creating a project
You learned how to create a Wear project in the previous chapter. We will be following a similar process with a slightly different configuration. In this project, we will choose Mobile and Wear app templates and extend them with further improvements in a later chapter.
Create a project called Wear-note-1:

- Your package address should be unique and should follow the reverse domain standard as Android standard development practices recommend it (for instance, com.yourname.projectname).
- Select a convenient project location depending on your accessibility.
- Select the form factors for your application to work on. Here, in this prompt, we will select both the Wear and mobile projects and select the most recent stable API 25 Nougat for the mobile and Wear projects:

- In the following prompt, we will be adding Activity for the mobile application; we shall choose the appropriate template from the prompt. For the scope of this project, let's select Empty Activity.

- In the next prompt, we shall give a name for the mobile application activity.

The previous prompt generates an Hello World application for mobile and generates all the necessary code stub.
- This prompt allows the user to choose a different set of Wear templates. Let the default selected template be the Wear application code stub, Always On Wear Activity:

- In the following prompt, give a suitable name for your Wear activity.

- On successful project creation, all the necessary gradle dependencies and the Java code stubs will be created. Android Studio will have the following configuration:

- wear Project
- Gradle Module: mobile
- Gradle Module: wear
The gradle module for mobile should include the Wear project in its dependency:
wearApp project(':wear')
The complete gradle module for mobile looks as follows:
build.gradle (Module: mobile)
apply plugin: 'com.android.application' android { compileSdkVersion 25 buildToolsVersion "25.0.2" defaultConfig { applicationId "com.ashok.packt.wear_note_1" minSdkVersion 25 targetSdkVersion 25 versionCode 1 versionName "1.0" testInstrumentationRunner
"android.support.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-
android.txt'), 'proguard-rules.pro' } } } dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) androidTestCompile('com.android.support.test.espresso:espresso-
core:2.2.2', { exclude group: 'com.android.support', module: 'support-
annotations' }) wearApp project(':wear') compile 'com.google.android.gms:play-services:10.0.1' compile 'com.android.support:appcompat-v7:25.1.1' testCompile 'junit:junit:4.12' }
In the gradle module for Wear, we can include wear-specific gradle dependencies:
compile 'com.google.android.support:wearable:2.0.0' compile 'com.google.android.gms:play-services-wearable:10.0.1' provided 'com.google.android.wearable:wearable:2.0.0'
The complete gradle module for wear looks as follows:
apply plugin: 'com.android.application' android { compileSdkVersion 25 buildToolsVersion "25.0.2" defaultConfig { applicationId "com.ashok.packt.wear_note_1" minSdkVersion 25 targetSdkVersion 25 versionCode 1 versionName "1.0" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-
android.txt'), 'proguard-rules.pro' } } } dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) compile 'com.google.android.support:wearable:2.0.0' compile 'com.google.android.gms:play-services-wearable:10.0.1' provided 'com.google.android.wearable:wearable:2.0.0' }
Now, we are all set to get started on making a few corrections and code removal in the generated code stub for the note application.
In the generated code stub for the Wear application, MainActivity, except the onCreate() method, removes all the other methods and codes:
Under the res directory in the project section, go to the layout directory, select the activity_main.xml file, and remove the TextView components generated. Keep the BoxInsetLayout container. The complete XML will look as follows:
<?xml version="1.0" encoding="utf-8"?> <android.support.wearable.view.BoxInsetLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/container" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.ashok.packt.wear_note_1.MainActivity" tools:deviceIds="wear"> </android.support.wearable.view.BoxInsetLayout>
Now, we are all set to write the note application. Before we jump into writing the code, it is very important to understand the lifecycle of an activity.