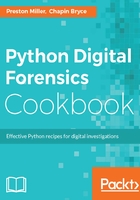
How it works...
First, we import the required libraries to handle argument parsing, sleeping the script, and taking screenshots:
from __future__ import print_function
import argparse
from multiprocessing import freeze_support
import os
import sys
import time
try:
import pyscreenshot
import wx
except ImportError:
print("[-] Install wx and pyscreenshot to use this script")
sys.exit(1)
This recipe's command-line handler takes two positional arguments, OUTPUT_DIR and INTERVAL, which represent the desired output path and the interval between screenshots, respectively. The optional total argument can be used to impose an upper limit on the number of screenshots that should be taken. Note that we specify the type for both INTERVAL and total arguments as integers. After validating that the output directory exists, we pass these inputs to the main() method:
if __name__ == "__main__":
# Command-line Argument Parser
parser = argparse.ArgumentParser(
description=__description__,
epilog="Developed by {} on {}".format(
", ".join(__authors__), __date__)
)
parser.add_argument("OUTPUT_DIR", help="Desired Output Path")
parser.add_argument(
"INTERVAL", help="Screenshot interval (seconds)", type=int)
parser.add_argument(
"-total", help="Total number of screenshots to take", type=int)
args = parser.parse_args()
if not os.path.exists(args.OUTPUT_DIR):
os.makedirs(args.OUTPUT_DIR)
main(args.OUTPUT_DIR, args.INTERVAL, args.total)
The main() function creates an infinite while loop and starts incrementing a counter by one for each screenshot taken. Following that, the script sleeps for the provided interval before using the pyscreenshot.grab() method to capture a screenshot. With the screenshot captured, we create the output filename and use the screenshot object's save() method to save it to the output location. That's really it. We print a status message notifying the user about this and then check whether the total argument was provided and whether the counter is equal to it. If it is, the while loop is exited, but otherwise, it continues forever. As a word of caution/wisdom, if you choose not to provide a total limit, make sure to stop the script manually once you have completed your review. Otherwise, you may come back to an ominous blue screen and full hard drive:
def main(output_dir, interval, total):
i = 0
while True:
i += 1
time.sleep(interval)
image = pyscreenshot.grab()
output = os.path.join(output_dir, "screenshot_{}.png").format(i)
image.save(output)
print("[+] Took screenshot {} and saved it to {}".format(
i, output_dir))
if total is not None and i == total:
print("[+] Finished taking {} screenshots every {} "
"seconds".format(total, interval))
sys.exit(0)
With the screenshotting script running every five seconds and storing the pictures in the folder of our choice, we can see the following output, as captured in the following screenshot:
