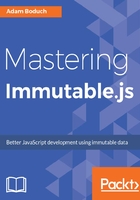
上QQ阅读APP看书,第一时间看更新
Chaining collection removal methods
If you have more than one value to remove from a collection, you can chain together removal method calls, as follows:
const myList = List.of(1, 2, 3, 4);
const myMap = Map.of(
'one', 1, 'two', 2,
'three', 3, 'four', 4,
'five', 5, 'six', 6
);
const myChangedList = myList
.remove(1)
.remove(1);
const myChangedMap = myMap
.remove('six')
.removeAll(['five', 'four', 'three']);
console.log('myList', myList.toJS());
// -> myList [ 1, 2, 3, 4 ]
console.log('myMap', myMap.toJS());
// -> myMap { one: 1, two: 2, three: 3, four: 4, five: 5, six: 6 }
console.log('myChangedList', myChangedList.toJS());
// -> myChangedList [ 1, 4 ]
console.log('myChangedMap', myChangedMap.toJS());
// -> myChangedMap { one: 1, two: 2 }
There are two identical removal calls to the list—remove(1). What's up with that? Chaining removal calls to remove list items can be tricky because removing one value causes every value to the right of it to change its index. At first, the index 1 points to the value 2. Once it's removed, the index 1 points to the value 3.
With maps, you have a couple of options for removing more than one key-value pair. You can chain together calls to remove(), or you can pass multiple keys to the removeAll() method.