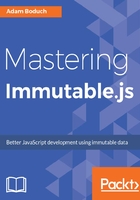
Updating list values
When the new value of a list item depends on the current value, you can use the update() method instead of set(). It takes a function that gets the current value as an argument and the return value is used as the new value, as shown here:
const myList = List.of(
Map.of('total', 0, 'step', 5),
Map.of('total', 5, 'step', 10)
);
const increment = map => map.set(
'total',
map.get('total') + map.get('step')
);
const myChangedList = myList
.update(0, increment)
.update(1, increment);
console.log('myList', myList.toJS());
// -> myList [ { total: 0, step: 5 }, { total: 5, step: 10 } ]
console.log('myChangedList', myChangedList.toJS());
// -> myChangedList [ { total: 5, step: 5 }, { total: 15, step: 10 } ]
Each value in this list is a map with two keys: total and step. The increment() function updates the total value based on its current value and the step value. The function then returns the new map that's created by calling set(). If you know the index of the list value that you want to update, you can pass the increment() function to update(), along with the index. In this example, I've updated both list items.
We could have achieved the same result by calling set() on the list, but this would involve manually getting the value from the list first, since we depend on it. Using update() is simpler because the value that we need is given to us as a function argument.