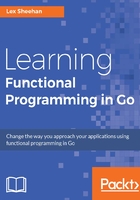
上QQ阅读APP看书,第一时间看更新
Generics
Parametric polymorphism means generics. A generic function or a data type can be written to handle any data value using the same logic, without having to cast the value to a specific data type. This greatly improves code reuse.
The following is a C# code example of a generic IsEqual implementation. The generic IsEqual function will accept any type (that implements Equals). We pass IsEqual integers and strings by simply indicating the type T during runtime, at the moment IsEqual is executed:
namespace Generics
{
private static void Main() {
if(Compute<int>.IsEqual(2, 2)) {
Console.WriteLine("2 isEqualTo 2");
}
if(!Compute<String>.IsEqual("A", "B")) {
Console.WriteLine("A is_NOT_EqualTo B");
}
}
public class Compute<T> {
public static bool IsEqual(T Val1, T Val2) {
return Val1.Equals(Val2);
}
}
}
Currently, to do this in Go, we will have to use an empty interface and perform a type conversion. It's type conversion that will cause the performance hit that usually makes this sort of generics handling in Go impractical.