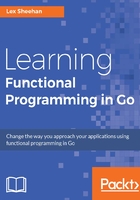
Function composition
Function composition is what happens when we combine functions. The output of one function is the the input of the next function. We can use objects and morphisms of category theory to help us get the order right. Take the following diagram for example...
>
We see that we can combine our functions f and g to get from A to B to C. Note that the order matters. We must first go from A to B via f and then from B to C via g.
We express this with the following notation (f.g)(x). That reads, f-compose-g with input x. This expression equals g(f(x)), which reads f of x of g. So (f.g)(x) == g(f(x)).
This is what the compose function looks like in Go:
func Compose(f StrFunc, g StrFunc) StrFunc {
return func(s string) string {
return g(f(s))
}
}
Where StrFunc is defined as:
type StrFunc func(string) string
In our main.go, we define our f and g functions, recognize and emphasize, respectively:
func main() {
var recognize = func(name string) string {
return fmt.Sprintf("Hey %s", name)
}
var emphasize = func(statement string) string {
return fmt.Sprintf(strings.ToUpper(statement) + "!")
}
We compose f and g as follows:
var greetFoG = Compose(recognize, emphasize)
fmt.Println(greetFoG("Gopher"))
The following is the output:
HEY GOPHER!
Note that order matters. What happens if we flip the order of f and g and then compose?
var greetGoF = Compose(emphasize, recognize)
fmt.Println(greetGoF("Gopher"))
The following is the output:
Hey GOPHER!