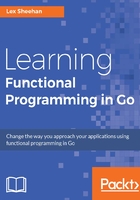
上QQ阅读APP看书,第一时间看更新
Itertools
Itertools is a Go package that provides many of the same high-order functions from the Python standard library.
Next, we see the different types of high-order functions provided by Itertools. High-order functions provide the vocabulary for the declarative coding style.
Infinite iterator creators:
- Count(i): Infinite count from i
- Cycle(iter): Infinite cycling of iter (requires memory)
- Repeat(element [, n]): Repeat the element n times (or infinitely)
Iterator destroyers:
- Reduce(iter, reducer, memo): Reduce (or Foldl) across the iterator
- List(iter): Create a list from the iterator
Iterator modifiers:
- Chain(iters...): Chain together multiple iterators.
- DropWhile(predicate, iter): Drop elements until predicate(el) == false.
- TakeWhile(predicate, iter): Take elements until predicate(el) == false.
- Filter(predicate, iter): Filter out elements when predicate(el) == false.
- FilterFalse(predicate, iter): Filter out elements when predicate(el) == true.
- Slice(iter, start[, stop[, step]]): Drop elements until the start (zero-based index). Stop upon stop (exclusive) unless not given. Step is 1 unless given.
More iterator modifiers:
- Map(mapper func(interface{}) interface{}, iter): Map each element to mapper(el).
- MultiMap(multiMapper func(interface{}...)interface{}, iters...): Map all the iterators as variadic arguments to multiMaper(elements...); stop at the shortest iterator.
- MultiMapLongest(multiMapper func(interface{}...)interface{}, iters...): Same as MultiMap, except that here you need to stop at the longest iterator. Shorter iterators are filled with nil after they are exhausted.
- Starmap(multiMapper func(interface{}...)interface{}, iter): If iter is an iterator of []interface{}, then expand it to multiMapper.
- Zip(iters...): Zip multiple iterators together.
- ZipLongest(iters...): Zip multiple iterators together. Take the longest; shorter ones are appended with nil.
- Tee(iter, n): Split an iterator into n equal versions.
- Tee2(iter): Split an iterator into two equal versions.