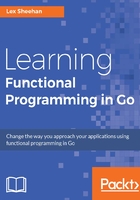
上QQ阅读APP看书,第一时间看更新
Map function
Let's create a Map function to transform the contents of a Collection.
First, let's define Object to be the empty interface type and create a Collection type to be a slice of objects:
package main
import "fmt"
type Object interface{}
type Collection []Object
func NewCollection(size int) Collection {
return make(Collection, size)
}
The NewCollection function creates a new instance of the collection with the given size:
type Callback func(current, currentKey, src Object) Object
The Callback type is a first-class function type that returns the calculated result:
func Map(c Collection, cb Callback) Collection {
if c == nil {
return Collection{}
} else if cb == nil {
return c
}
result := NewCollection(len(c))
for index, val := range c {
result[index] = cb(val, index, c)
}
return result
}
The Map function returns a new collection where every element is the result of calling the Callback function.