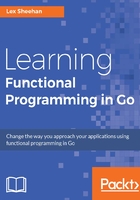
TCO recursive example
In our tail recursive function, our stack frames do not need to be preserved.
tSum :: [Integer] -> Integer
tSum lst = tSum lst 0 where
tSum (x:xs) i = tSum xs (i+x)
tSum [] i = i
The following diagram illustrates that unlike the previous example (rSum), no action needs to be taken in the context of a frame after tSum makes its recursive call. rSum created a stack frame for each member of the list. tSum only needs to create one stack frame, which it reuses.

TCO avoids creating a new stack frame when the last call in a recursion is the function itself. Go currently does not support TCO. What is the implication? Without TCO, we should avoid using recursion to process lists with a lot of elements, that is, over a few thousand; Otherwise, our program will likely run out of RAM and crash. Why not replace recursive functions with functions that implement imperative loops? In other words, what is the importance of recursion in functional programming?