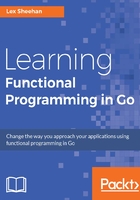
The Reduce function
Next, let's look at the Reduce function. Reduce is the Swiss army knife of HOFs. With a Reduce function, we can do anything that can be done with Filter or Map.
A Reduce function, also known as a fold, accumulate, aggregate, compress, or inject takes a seed value and applies the logic of the reducer function to the seed, and potentially multiple calls to itself to arrive at a result. Often, the reduce function will combine the data elements to return a single aggregated value, hence the term fold. So, we fold all of the data into a single result.
Back in main.go, we apply the ByMake filter to filter out all the cars that are not a Honda product. Then, we call the Reduce function to transform the collection of Honda vehicles into a collection of JSON strings:
PrintCars("Filter Honda, Reduce JSON",
cars.Filter(ByMake("Honda")).
Reduce(JsonReducer(cars), Collection{}))
The output of this will be as follows:
Filter Honda, Reduce JSON
-----------------------
car: {"car": {"make": "Honda", "model": " Accord"}}
car: {"car": {"make": "Honda", "model": " Accord ES2"}}
car: {"car": {"make": "Honda", "model": " CR-V"}}
The Reduce function is a method of the cars collection that accepts a Reducer function. Again, we see a for loop and recall, No TCO, No recursion. That's okay. So, the guts of our Reduce function is not pure. That's OK. It's still readable, performant, and safe; in the spirit of Go programming, it gets the job done:
func (cars Collection) Reduce(fn ReducerFunc, accumulator Collection) Collection {
var result = accumulator
for _, car := range cars {
result = append(fn(car, result))
}
return result
}
The second parameter Collection{} is the accumulator, which is the initial value assigned to the result. The Reducer function starts with the accumulator value, performs transformations on each item in the collection, and returns the result. This Reduce function provides the framework in which to perform a reduction, but it's the reducer function (fn) that does the heavy lifting. Note that we can pass any valid reducer function (fn) into the Reduce framework to get vastly different results.
Our JsonReducer function does the real work of transforming each item in the cars collection into a JSON string:
func JsonReducer(cars Collection) ReducerFunc {
return func(car string, cars Collection) Collection {
carJson := fmt.Sprintf("{"car": {"make": "%s", "model": "%s"}}", GetMake(car), GetModel(car))
cars = append(cars, carJson)
return cars
}
}
Reduce is an HOF function that takes a function. JsonReducer is an HOF function that returns a function.