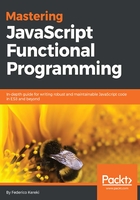
Detecting Ajax
Let's go back a bit to the times when Ajax started to appear. Given those different browsers implemented Ajax calls in distinct fashions, you would always have to code around those differences:
function getAjax() {
let ajax = null;
if (window.XMLHttpRequest) {
// modern browser? use XMLHttpRequest
ajax = new XMLHttpRequest();
} else if (window.ActiveXObject) {
// otherwise, use ActiveX for IE5 and IE6
ajax = new ActiveXObject("Microsoft.XMLHTTP");
} else {
throw new Error("No Ajax support!");
}
return ajax;
}
This worked but implied that you would re-do the Ajax check for each and every call -- even though the results of the test wouldn't ever change. There's a more efficient way to do so, and it has to do with using functions as first-class objects. We could define two different functions, test for the condition only once, and then assign the correct function to be used later:
(function initializeGetAjax() {
let myAjax = null;
if (window.XMLHttpRequest) {
// modern browsers? use XMLHttpRequest
myAjax = function() {
return new XMLHttpRequest();
};
} else if (window.ActiveXObject) {
// it's ActiveX for IE5 and IE6
myAjax = function() {
new ActiveXObject("Microsoft.XMLHTTP");
};
} else {
myAjax = function() {
throw new Error("No Ajax support!");
};
}
window.getAjax = myAjax;
})();
This piece of code shows two important concepts. First, we can dynamically assign a function: when this code runs, window.getAjax (that is, the global getAjax variable) will get one of three possible values, according to the current browser. When you later call getAjax() in your code, the right function will execute, without needing to do any further browser detection tests.
The second interesting idea is that we define the initializeGetAjax function, and immediately run it -- this pattern is called IIFE, standing for Immediately Invoked Function Expression. The function runs, but cleans after itself, for all its variables are local, and won't even exist after the function runs. We'll see more about this later.