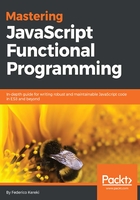
Callbacks, promises, and continuations
Probably the most used example of functions passed as first-class objects has to do with callbacks and promises. In Node.JS, reading a file is accomplished asynchronically with something like this:
const fs = require("fs");
fs.readFile("someFile.txt", (err, data) => {
if (err) {
console.error(err); // or throw an error, or otherwise handle the problem
} else {
console.log(data.toString());
}
});
The readFile() function requires a callback, which in this example is just an anonymous function, that gets called when the file reading operation is finished.
With a more modern programming style, you would use promises or async/await.. For instance, when doing an Ajax web service call, using the more modern fetch() function, you could write something along the lines of the following code:
fetch("some/remote/url")
.then(data => {
// Do some work with the returned data
})
.catch(error => {
// Process all errors here
});
Note that if you had defined appropriate processData(data) and processError(error) functions, the code could have been shortened to fetch("some/remote/url").then(processData).catch(processError) along the lines that we saw previously.