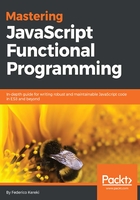
Solution #7- use a local flag
We can go back to the idea of using a flag, but instead of making it global (which was our main objection) we can use a Immediately Invoked Function Expression (IIFE): we'll see more on this in Chapter 3, Starting Out with Functions - A Core Concept, and in Chapter 11, Implementing Design Patterns - The Functional Way. With this, we can use a closure, so clicked will be local to the function, and not visible anywhere else:
var billTheUser = (clicked => {
return (some, sales, data) => {
if (!clicked) {
clicked = true;
window.alert("Billing the user...");
// actually bill the user
}
};
})(false);
See how clicked gets its initial false value, from the call at the end.
This solution is along the lines of the global variable solution, but using a private, local variable is an enhancement. About the only objection we could find, is that you'll have to rework every function that needs to be called only once, to work in this fashion. (And, as we'll see in the following section, our FP solution is similar in some ways to it.) OK, it's not too hard to do, but don't forget the Don't Repeat Yourself (D.R.Y) advice!