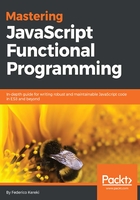
Spread
The spread operator (see https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Operators/Spread_operator) lets you expand an expression in places where you would otherwise require multiple arguments, elements, or variables. For example, you can replace arguments in a function call:
const x = [1, 2, 3];
function sum3(a, b, c) {
return a + b + c;
}
const y = sum3(...x); // equivalent to sum3(1,2,3)
console.log(y); // 6
You can also create or join arrays:
const f = [1, 2, 3];
const g = [4, ...f, 5]; // [4,1,2,3,5]
const h = [...f, ...g]; // [1,2,3,4,1,2,3,5]
It works with objects too:
const p = { some: 3, data: 5 };
const q = { more: 8, ...p }; // { more:8, some:3, data:5 }
You can also use it to work with functions that expect separate parameters, instead of an array. Common examples of this would be Math.min() and Math.max():
const numbers = [2, 2, 9, 6, 0, 1, 2, 4, 5, 6];
const minA = Math.min(...numbers); // 0
const maxArray = arr => Math.max(...arr);
const maxA = maxArray(numbers); // 9
You can also write the following equation. The .apply() method requires an array of arguments, but .call() expects inpidual arguments:
someFn.apply(thisArg, someArray) === someFn.call(thisArg, ...someArray);
If you have problems remembering what arguments are required by .apply() and .call(), this mnemonic may help: A is for array, and C is for comma. See https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function/apply and https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function/call for more information.
Using the spread operator helps write shorter, more concise code, and we will be taking advantage of it.