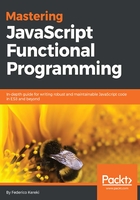
Closures
Closures are a way to implement data hiding (with private variables), which leads to modules and other nice features. The key concept is that when you define a function, it can refer to not only its own local variables, but also to everything outside of the context of the function:
function newCounter() {
let count = 0;
return function() {
count++;
return count;
};
}
const nc = newCounter();
console.log(nc()); // 1
console.log(nc()); // 2
console.log(nc()); // 3
Even after newCounter exits, the inner function still has access to count, but that variable is not accessible to any other parts of your code.
This isn't a very good example of FP -- a function (nc(), in this case) isn't expected to return different results when called with the same parameters!
We'll find several uses for closures: among others, memoization (see chapter 4, Behaving Properly - Pure Functions, and chapter 6, Producing Functions - Higher-Order Functions) and the module pattern (see chapter 3, Starting Out with Functions - A Core Concept, and chapter 11, Implementing Design Patterns - The Functional Way).