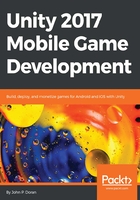
上QQ阅读APP看书,第一时间看更新
Putting it all together
With all of the stuff we've been talking about, we can now have the final version of the script, which looks like the following:
using UnityEngine;
/// <summary>
/// Responsible for moving the player automatically and
/// reciving input.
/// </summary>
[RequireComponent(typeof(Rigidbody))]
public class PlayerBehaviour : MonoBehaviour
{
/// <summary>
/// A reference to the Rigidbody component
/// </summary>
private Rigidbody rb;
[Tooltip("How fast the ball moves left/right")]
public float dodgeSpeed = 5;
[Tooltip("How fast the ball moves forwards automatically")]
[Range(0, 10)]
public float rollSpeed = 5;
/// <summary>
/// Use this for initialization
/// </summary>
void Start ()
{
// Get access to our Rigidbody component
rb = GetComponent<Rigidbody>();
}
/// <summary>
/// Update is called once per frame
/// </summary>
void Update ()
{
// Check if we're moving to the side
var horizontalSpeed = Input.GetAxis("Horizontal") *
dodgeSpeed;
// Apply our auto-moving and movement forces
rb.AddForce(horizontalSpeed, 0, rollSpeed);
}
}
I hope that you also agree that this makes the code easier to understand and better to work with.