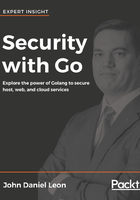
上QQ阅读APP看书,第一时间看更新
Function
Functions are defined with the func keyword. Functions can have multiple parameters. All parameters are positional and there are no named parameters. Go supports variadic parameters allowing for an unknown number of parameters. Functions are first-class citizens in Go, and can be used anonymously and returned as a variable. Go also supports multiple return values from a function. The underscore can be used to ignore a return variable.
All of these examples are demonstrated in the following code source:
package main
import "fmt"
// Function with no parameters
func sayHello() {
fmt.Println("Hello.")
}
// Function with one parameter
func greet(name string) {
fmt.Printf("Hello, %s.\n", name)
}
// Function with multiple params of same type
func greetCustom(name, greeting string) {
fmt.Printf("%s, %s.\n", greeting, name)
}
// Variadic parameters, unlimited parameters
func addAll(numbers ...int) int {
sum := 0
for _, number := range numbers {
sum += number
}
return sum
}
// Function with multiple return values
// Multiple values encapsulated by parenthesis
func checkStatus() (int, error) {
return 200, nil
}
// Define a type as a function so it can be used
// as a return type
type greeterFunc func(string)
// Generate and return a function
func generateGreetFunc(greeting string) greeterFunc {
return func(name string) {
fmt.Printf("%s, %s.\n", greeting, name)
}
}
func main() {
sayHello()
greet("NanoDano")
greetCustom("NanoDano", "Hi")
fmt.Println(addAll(4, 5, 2, 3, 9))
russianGreet := generateGreetFunc("Привет")
russianGreet("NanoDano")
statusCode, err := checkStatus()
fmt.Println(statusCode, err)
}