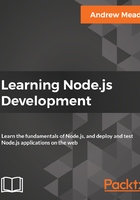
Reading out the content in the file
The first step to print the title is to use a method we haven't used yet. We'll use the read method available on the filesystem module to read the contents. Let's make a variable called noteString. The noteString variable will be set equal to fs.readFileSync.
Now, readFileSync is similar to writeFileSync except that it doesn't take the text content, since it's getting the text content back for you. In this case, we'll just specify the first argument, which is the filename, notes.JSON:
var noteString = fs.readFileSync('notes.json');
Now that we have the string, it will be your job to take that string, use one of the preceding methods, and convert it back into an object. You can call that variable note. Next up, the only thing left to do is to test whether things are working as expected, by printing with the help of console.log(typeof note). Then, below this, we'll use console.log to print the title, note.title:
// note
console.log(typeof note);
console.log(note.title);
Now, over in Terminal, you can see (refer to the following screenshot) that I have saved the file in a broken state and it crashed, and that's expected when you're using nodemon:

To resolve this, the first thing I'll do is fill out the originalNoteString variable, which we had commented out earlier. It will now be a variable called originalNoteString, and we'll set it equal to the return value from JSON.stringify.
Now, we know JSON.stringify takes our regular object and it converts the object into a string. In this case, we'll take the originalNote object and convert it into a string. The next line, which we already have filled out, will save that JSON value into the notes.JSON file. Then we will read that value out:
var originalNoteString = JSON.stringify(originalNote);
The next step will be to create the note variable. The note variable will be set equal to JSON.parse.
The JSON.parse method takes the string JSON and converts it back into a regular JavaScript object or array, depending on whatever you save. Here we will pass in noteString, which we'll get from the file:
var note = JSON.parse(noteString);
With this in place, we are now done. When I save this file, nodemon will automatically restart and we would expect to not see an error. Instead, we expect that we'll see the object type as well as the note title. Right inside Terminal, we have object and Some title printing to the screen:

With this in place, we've successfully completed the challenge. This is exactly how we will save our notes.
When someone adds a new note, we'll use the following code to save it:
var originalNote = {
title: 'Some title',
body: 'Some body'
};
var originalNoteString = JSON.stringify(originalNote);
fs.writeFileSync('notes.json', originalNoteString);
When someone wants to read their note, we'll use the following code to read it:
var noteString = fs.readFileSync('notes.json');
var note = JSON.parse(noteString);
console.log(typeof note);
console.log(note.title);
Now, what if someone wants to add a note? This will require us to first read all of the notes, then modify the notes array, and then use the code (refer to the previous code block) to save the new array back into the filesystem.
If you open up that notes.JSON file, you can see right here that we have our JSON code inside the file:

.json is actually a file format that's supported by most text editors, so I actually already have some nice syntax highlighting built in. Now, in the next section, we'll be filling out the addNote function using the exact same logic that we just used inside of this section.