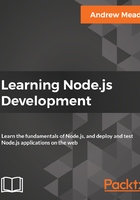
Converting a string back to an object
To convert the string back to object, we'll use the opposite of JSON.stringify, which is JSON.parse. Let's make a variable to store the result. I'll create a person variable and it will be set equal to JSON.parse, passing in as the one and only argument the string you want to parse, in this case, the person string, which we defined earlier:
var person = JSON.parse(personString);
Now, this variable takes your JSON and converts it from a string back into its original form, which could be an array or an object. In our case, it converts it back into an object, and we have the person variable as an object, as shown in the preceding code. Also, we can prove that it's an object using the typeof operator. I'll use console.log twice, just like we did previously.
First up, we'll print typeof person, and then we'll print the actual person variable, console.log(person):
console.log(typeof person);
console.log(person);
With this in place, we can now rerun the command in Terminal; I'll actually start nodemon and pass in json.js:
nodemon json.js
As shown in the following code output, you can now see that we're working with an object, which is great, and we have our regular object:

We know that Andrew is an object because it's not wrapped in double quotes; the values don't have any quotes, and we use single quotes for Andrew, which is valid in JavaScript, but it's not valid in JSON.
This is the entire process of taking an object, converting it to a string, and then taking the string and converting it back into the object, and this is exactly what we'll do in the notes app. The only difference is that we'll be taking the following string and storing it in a file, then later on, we'll be reading that string from the file using JSON.parse to convert it back to an object, as shown in the following code block:
// var obj = {
// name: 'Andrew'
// };
// var stringObj = JSON.stringify(obj);
// console.log(typeof stringObj);
// console.log(stringObj);
var personString = '{"name": "Andrew","age": 25}';
var person = JSON.parse{personString};
console.log(typeof person);
console.log(person);