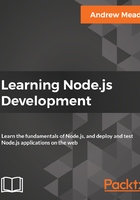
The remove command
Now, since the read command is working, we can move on to the last one, which is the remove command. Here, I'll call notes.removeNote, passing in the title, which as we know is available in argv.title:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const yargs = require('yargs');
const notes = require('./notes.js');
const argv = yargs.argv;
var command = process.argv[2];
console.log('Command:', command);
console.log('Yargs', argv);
if (command === 'add') {
notes.addNote(argv.title, argv.body);
} else if (command === 'list') {
notes.getAll();
} else if (command === 'read') {
notes.getNote(argv.title);
} else if (command === 'remove') {
notes.removeNote(argv.title);
} else {
console.log('Command not recognized');
}
Next up, we'll define the removeNote function over inside of our notes API file, right below the getNote variable:
var removeNote = (title) => { console.log('Removing note', title); };
Now, removeNote will work much the same way as getNote. All it needs is the title; it can use this information to find the note and remove it from the database. This will be an arrow function that takes the title argument.
In this case, we'll print the console.log statement, Removing note; then, as the second argument, we'll simply print title back to the screen to make sure that it's going through the process successfully. This time around, we'll export our removeNote function; we'll define it using the ES6 syntax:
module.exports = {
addNote,
getAll,
getNote,
removeNote
};
The last thing to do is test it and make sure it works. We can reload the last command using the up arrow key. We change read to remove, and that is all we need to do. We're still passing in the title argument, which is great, because that is what remove needs:
node app.js remove --title accounts
When I run this command, we get exactly what we expected. Removing note prints to the screen, as shown in the following code output, and then we get the title of the note that we're supposed to be removing, which is accounts:

This looks great! That is all it takes to use yargs to parse your arguments.
With this, we now have a place to define all of that functionality, for saving, reading, listing, and removing notes.