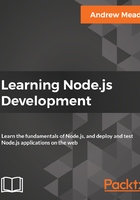
Solution to the exercise
For the solution, the first thing I'll do is to add an else if for read. I'll open and close my curly braces and hit enter right in the middle so everything gets formatted correctly.
In the else if statement, I'll check whether the command variable equals the string read, as shown here:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command: ', command);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else if () {
} else {
console.log('Command not recognized');
}
For now, we'll use console.log to print Reading note:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command: ', command);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else if (command === 'read') {
} else {
console.log('Command not recognized');
}
The next thing you need to do is add an else if clause that checks whether the command equals remove. In the else if, I'll open and close my condition and hit enter just as I did in the previous else if clause; this time, I'll add if the command equals remove, we want to remove the note. And in that case, all we'll do is to use console.log to print Reading note, as shown in the following code:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command: ', command);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else if (command === 'read') {
console.log('Reading note');
} else {
console.log('Command not recognized');
}
And with this in place, we are done. If we refer to the code block, we've added two new commands we can run over in the Terminal, and we can test those:
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else if (command === 'read') {
console.log('Reading note');
} else {
console.log('Command not recognized');
}
First up, I'll run node app.js with the read command, and Reading note shows up:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
var command = process.argv[2];
console.log('Command: ', command);
if (command === 'add') {
console.log('Adding new note');
} else if (command === 'list') {
console.log('Listing all notes');
} else if (command === 'read') {
console.log('Reading note');
} else if (command == 'remove') {
console.log('Removing note');
} else {
console.log('Command not recognized');
}
Then I'll rerun the command; this time, I'll be using remove. And when I do that, Removing note prints to the screen, as shown in this screenshot:

I'll wrap up my testing using a command that doesn't exist, and when I run that, you can see Command not recognized shows up.