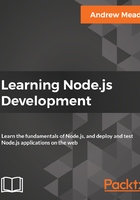
Executing nodemon
Nodemon will get executed as Node did, where we type the command and then we type the file we want to start. In our case, app.js is the root of our project. When you run it, you'll see a few things, as shown here:

We'll see a combination of our app's output, along with nodemon logs that show you what's happening. As shown in the preceding code, you can see the version nodemon is using, the files it's watching, and the command it actually ran. Now, at this point, it's waiting for more changes; it already ran through the entire app and it'll keep running until another change happens or until you shut it down.
Inside Atom, we'll make a few changes to our app. Let's get started by changing Gary to Mike in app.js, and then we'll change the filteredArray variable to var filteredArray = _.uniq(['Mike']), as shown in the following code:
console.log('Starting app.js');
const fs = require('fs');
const os = require('os');
const _ = require('lodash');
const notes = require('./notes.js');
// console.log(_.isString(true));
// console.log(_.isString('Gary'));
var filteredArray = _.uniq(['Mike']);
console.log(filteredArray);
Now, I'll be saving the file. In the Terminal window, you can see the app automatically restarted, and within a split second, the new output is shown on the screen:

As shown in the preceding screenshot, we now have our array with one item of string, Mike. And this is the real power of nodemon.
You can create your applications and they will automatically restart over in the Terminal, which is super useful. It'll save you a ton of time and a ton of headaches. You won't have to switch back and forth every time you make a small tweak. This also prevents a ton of errors where you are running a web server, you make a change, and you forget to restart the web server. You might think your change didn't work as expected because the app is not working as expected, but in reality, you just never restarted the app.
For the most part, we will be using nodemon throughout the book since it's super useful. It's only used for development purposes, which is exactly what we're doing on our local machine. Now, we'll move forward and start exploring how we can get input from the user to create our notes application. That will the topic of the next few sections.
Before we get started, we should clean up a lot of the code we've already written in this section. I'll remove all of the commented-out code in app.js. Then, I'll simply remove os, where we have fs, os and lodash, since we'll not be using it throughout the project. I'll also be adding a space between the third-party and Node modules and the files I've written, which are as follows:
console.log('Starting app.js');
const fs = require('fs');
const _ = require('lodash');
const notes = require('./notes.js');
I find this to be a good syntax that makes it a lot easier to quickly scan for either third-party or Node modules, or the modules that I've created and required.
Next up, over in notes.js, we'll remove the add function; this was only added for demonstration purposes, as shown in the following figure. Then we can save both the notes.js and app.js files, and nodemon will automatically restart:
console.log('Starting notes.js');
module.exports.addNote = () => {
console.log('addNote');
return 'New note';
};
module.exports.add = (a, b) => {
return a + b;
};
Now we can remove the greetings.txt file. That was used to demonstrate how the fs module works, and since we already know how it works, we can wipe that file. And last but not least, we can always shut down nodemon using Ctrl + C. Now we're back at the regular Terminal.
And with this in place, now we should move on, figuring out how we can get input from the user, because that's how users can create notes, remove notes, and fetch their notes.