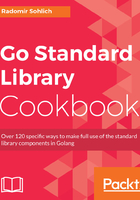
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open the console and create the folder chapter03/recipe05.
- Navigate to the directory.
- Create the format.go file with the following content:
package main
import (
"fmt"
)
var integer int64 = 32500
var floatNum float64 = 22000.456
func main() {
// Common way how to print the decimal
// number
fmt.Printf("%d \n", integer)
// Always show the sign
fmt.Printf("%+d \n", integer)
// Print in other base X -16, o-8, b -2, d - 10
fmt.Printf("%X \n", integer)
fmt.Printf("%#X \n", integer)
// Padding with leading zeros
fmt.Printf("%010d \n", integer)
// Left padding with spaces
fmt.Printf("% 10d \n", integer)
// Right padding
fmt.Printf("% -10d \n", integer)
// Print floating
// point number
fmt.Printf("%f \n", floatNum)
// Floating-point number
// with limited precision = 5
fmt.Printf("%.5f \n", floatNum)
// Floating-point number
// in scientific notation
fmt.Printf("%e \n", floatNum)
// Floating-point number
// %e for large exponents
// or %f otherwise
fmt.Printf("%g \n", floatNum)
}
- Execute the code by running go run format.go in the main Terminal.
- You will see the following output:

- Create the file localized.go with the following content:
package main
import (
"golang.org/x/text/language"
"golang.org/x/text/message"
)
const num = 100000.5678
func main() {
p := message.NewPrinter(language.English)
p.Printf(" %.2f \n", num)
p = message.NewPrinter(language.German)
p.Printf(" %.2f \n", num)
}
- Execute the code by running go run localized.go in the main Terminal.
- You will see the following output:
