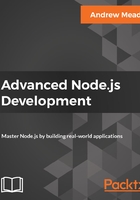
Adding data to the database
Inside of Atom, before our call to db.close, we're going to insert a new record into a collection. This is going to be the Todo application. We're going to have two collections in this app:
- a Todos collection
- a Users collection
We can go ahead and start adding some data to the Todos collection by calling db.collection. The db.collection method takes the string name for the collection you want to insert into as its only argument. Now, like the actual database itself, you don't need to create this collection first. You can simply give it a name, like Todos, and you can start inserting into it. There is no need to run any command to create it:
db.collection('Todos')
Next, we're going to use a method available in our collection called insertOne. The insertOne method lets you insert a new document into your collection. It takes two arguments:
- The first one is going to be an object. This is going to store the various key-value pairs we want to have in our document.
- The second one is going to be a callback function. This callback function will get fired when things either fail or go well.
You're going to get an error argument, which may or may not exist, and you'll also get the result argument, which is going to be provided if things went well:
const MongoClient = require('mongodb').MongoClient; MongoClient.connect('mongodb://localhost:27017/TodoApp', (err, client) => { if(err){ console.log('Unable to connect to MongoDB server'); } console.log('Connected to MongoDB server');
const db = client.db('TodoApp');
db.collection('Todos').insertOne({ text: 'Something to do', completed: false }, (err, result) => {
}); client.close(); });
Inside of the error callback function itself, we can add some code to handle the error, and then we'll add some code to print the object to the screen if it was added successfully. First up, let's add an error handler. Much like we have done previously, we're going to check if the error argument exists. If it does, then we'll simply print a message using the return keyword to stop the function from executing. Next, we can use console.log to print Unable to insert todo. The second argument I'm going to pass to the console.log is going to be the actual err object itself, so if someone's looking at the logs, they can see exactly what went wrong:
db.collection('Todos').insertOne({ text: 'Something to do', completed: false }, (err, result) => { if(err){ return console.log('Unable to insert todo', err); }
Next to our if statement, we can add our success code. In this case, all we're going to do is pretty-print something to the console.log screen, and then I'm going to call JSON.stringify, where we're going to go ahead and pass in result.ops. The ops attribute is going to store all of the docs that were inserted. In this case, we used insertOne, so it's just going to be our one document. Then, I can add my other two arguments, which are undefined for the filter function, and 2 for the indentation:
db.collection('Todos').insertOne({ text: 'Something to do', completed: false }, (err, result) => { if(err){ return console.log('Unable to insert todo', err); }
console.log(JSON.stringify(result.ops, undefined, 2)); });
With this in place, we can now go ahead and execute our file and see what happens. Inside of the Terminal, I'm going to run the following command:
node playground/ mongodb-connect.js
When I execute the command, we get our success message: Connected to MongoDB server. Then, we get an array of documents that were inserted:

Now as I mentioned, in this case we just inserted one document, and that shows up as shown in the preceding screenshot. We have the text property, which gets created by us; we have the completed property, which gets created by us; and we have the _id property, which gets automatically added by Mongo. The _id property is going to be the topic of the following section. We're going to talk in depth about what it is, why it exists and why it's awesome.
For now, we're going to go ahead and just note that it's a unique identifier. It's an ID given to just this document. That is all it takes to insert a document into your MongoDB database using Node.js. We can view this document inside of Robomongo. I'm going to right-click the connection, and click Refresh:

This reveals our brand new TodoApp database. If we open that up, we get our Collections list. We can then go into the Collections, view the documents, and what do we get? We get our one Todo item. If we expand it, we can see we have our _id, we have our text property, and we have our completed Boolean:

In this case, the Todo is not completed, so the completed value is false. Now, what I want you to do is add a new record into a collection. This is going to be your challenge for the section.