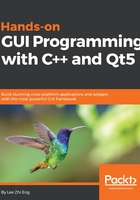
Creating our functional login page
Since we have learned how to connect our Qt application to the MariaDB/MySQL database system, it's time to continue working on the login page! In the previous chapter, we learned how to set up the GUI of our login page. However, it didn't have any functionality at all as a login page since it doesn't connect to the database and verify login credentials. Therefore, we will learn how to achieve that by empowering Qt's sql module.
Just to recap—this is what the login screen looks like:

The very first thing we need to do now is to name the widgets that are important in this login page, which are the Username input, Password input, and the Submit button. You can set these properties by selecting the widget and looking for the property in the property editor:

Then, set the echoMode of the password input as Password. This setting will hide the password visually by replacing it with dots:

After that, right-click on the Submit button and select Go to slot... A window will pop up and ask you which signal to use. Select clicked() and click OK:

A new function called on_loginButton_clicked() will be automatically added to the MainWindow class. This function will be triggered by Qt when the Submit button is pressed by the user, and thus you just need to write the code here to submit the username and password to the database for login verification. The signal and slots mechanism is a special feature provided by Qt which is used for communication between objects. When one widget is emitting a signal, another widget will be notified and will proceed to run a specific function that is designed to react to the particular signal.
Let's check out the code.
First, add in the sql keyword at your project (.pro) file:
QT += core gui
sql
Then, proceed and add the relevant headers to mainwindow.cpp:
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include <QtSql> #include <QSqlDatabase> #include <QSqlQuery> #include <QDebug> #include <QMessageBox>
Then, go back to mainwindow.cpp and add the following code to the on_loginButton_clicked() function:
void MainWindow::on_loginButton_clicked() { QString username = ui->userInput->text(); QString password = ui->passwordInput->text(); qDebug() << username << password; }
Now, click the Run button and wait for the application to start. Then, key in any random username and password, followed by clicking on the submit button. You should now see your username and password being displayed on the application output window in Qt Creator.
Next, we'll copy the SQL integration code we have written previously into mainwindow.cpp:
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); db = QSqlDatabase::addDatabase("QMYSQL"); db.setHostName("127.0.0.1"); db.setPort(3306); db.setDatabaseName("test"); db.setUserName("testuser"); db.setPassword("testpass"); if (db.open()) { qDebug() << "Connected!"; } else { qDebug() << "Failed to connect."; } }
Do note that I've used some random text for the database name, username, and password. Please make sure you enter the correct details here and that they match with what you've set in the database system.
One minor thing we have changed for the preceding code is that we only need to call db = QSqlDatabase::addDatabase("QMYSQL") in mainwindow.cpp without the class name as the declaration QSqlDatabase db has now been relocated to mainwindow.h:
private: Ui::MainWindow *ui; QSqlDatabase db;
Lastly, we add in the code that combines the username and password information with the SQL command, and send the whole thing to the database for execution. If there is a result that matches the login information, then it means that the login has been successful, otherwise, it means the login has failed:
void MainWindow::on_loginButton_clicked() { QString username = ui->userInput->text(); QString password = ui->passwordInput->text(); qDebug() << username << password; QString command = "SELECT * FROM user WHERE username = '" + username
+ "' AND password = '" + password + "' AND status = 0"; QSqlQuery query(db); if (query.exec(command)) { if (query.size() > 0) { QMessageBox::information(this, "Login success.", "You
have successfully logged in!"); } else { QMessageBox::information(this, "Login failed.", "Login
failed. Please try again..."); } } }
Click the Run button again and see what happens when you click the Submit button:

Hip hip hooray! The login page is now fully functional!