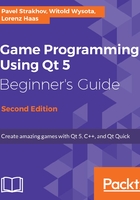
Showing specific areas of the scene
As soon as the scene's bounding rectangle exceeds the viewport's size, the view will show scroll bars. Besides using them with the mouse to navigate to a specific item or point on the scene, you can also access them by code. Since the view inherits QAbstractScrollArea, you can use all its functions for accessing the scroll bars; horizontalScrollBar() and verticalScrollBar() return a pointer to QScrollBar, and thus you can query their range with minimum() and maximum(). By invoking value() and setValue(), you get and can set the current value, which results in scrolling the scene.
However, normally, you do not need to control free scrolling inside the view from your source code. The normal task would be to scroll to a specific item. In order to do that, you do not need to do any calculations yourself; the view offers a pretty simple way to do that for you—centerOn(). With centerOn(), the view ensures that the item, which you have passed as an argument, is centered on the view unless it is too close to the scene's border or even outside. Then, the view tries to move it as far as possible on the center. The centerOn() function does not only take a QGraphicsItem item as argument; you can also center on a QPointF pointer or as a convenience on an x and y coordinate.
If you do not care where an item is shown, you can simply call ensureVisible() with the item as an argument. Then, the view scrolls the scene as little as possible so that the item's center remains or becomes visible. As a second and third argument, you can define a horizontal and vertical margin, which are both the minimum space between the item's bounding rectangle and the view's border. Both values have 50 pixels as their default value. Besides a QGraphicsItem item, you can also ensure the visibility of a QRectF element (of course, there is also the convenience function taking four qreal elements).
centerOn() and ensureVisible() only scroll the scene but do not change its transformation state. If you absolutely want to ensure the visibility of an item or a rectangle that exceeds the size of the view, you have to transform the scene as well. With this task, again the view will help you. By calling fitInView() with QGraphicsItem or a QRectF element as an argument, the view will scroll and scale the scene so that it fits in the viewport size.
As a second argument, you can control how the scaling is done. You have the following options:
Value | Description |
Qt::IgnoreAspectRatio |
The scaling is done absolutely freely regardless of the item's or rectangle's aspect ratio. |
Qt::KeepAspectRatio |
The item's or rectangle's aspect ratio is taken into account while trying to expand as far as possible while respecting the viewport's size. |
Qt::KeepAspectRatioByExpanding |
The item's or rectangle's aspect ratio is taken into account, but the view tries to fill the whole viewport's size with the smallest overlap. |
The fitInView() function does not only scale larger items down to fit the viewport, it also enlarges items to fill the whole viewport. The following diagram illustrates the different scaling options for an item that is enlarged (the circle on the left is the original item, and the black rectangle is the viewport):
