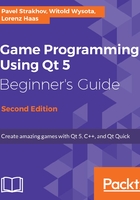
Time for action – Adding path items to the scene
Let's paint a few objects consisting of a large number of lines:
static const int SIZE = 100; static const int MARGIN = 10; static const int FIGURE_COUNT = 5; static const int LINE_COUNT = 500; for(int figureNum = 0; figureNum < FIGURE_COUNT; ++figureNum) { QPainterPath path; path.moveTo(0, 0); for(int i = 0; i < LINE_COUNT; ++i) { path.lineTo(qrand() % SIZE, qrand() % SIZE); } QGraphicsPathItem *item = scene.addPath(path); item->setPos(figureNum * (SIZE + MARGIN), 0); }
For each item, we first create a QPainterPath and set the current position to (0, 0). Then, we use the qrand() function to generate random numbers, apply the modulus operator (%) to produce a number from 0 to SIZE (excluding SIZE), and feed them to the lineTo() function that strokes a line from the current position to the given position and sets it as the new current position. Next, we use the addPath() convenience function that creates a QGraphicsPathItem object and adds it to the scene. Finally, we use setPos() to move each item to a different position in the scene. The result looks like this:

Using painter paths in this example is very efficient, because we avoided creating thousands of individual line objects on the heap. However, putting a large part of a scene in a single item may reduce the performance. When parts of the scene are separate graphics items, Qt can efficiently determine which items are not visible and skip drawing them.