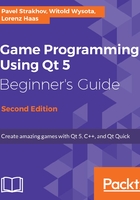
Standard items
In order to effectively use the framework, you need to know what graphics item classes it provides. It's important to identify the classes you can use to construct the desirable picture and resort to creating a custom item class, only if there is no suitable item or you need better performance. Qt comes with the following standard items that make your life as a developer much easier:
Standard item | Description |
QGraphicsLineItem |
Draws a line. You can define the line with setLine(const QLineF&). |
QGraphicsRectItem |
Draws a rectangle. You can define the rectangle's geometry with setRect(const QRectF&). |
QGraphicsEllipseItem |
Draws an ellipse or an ellipse segment. You can define the rectangle within which the ellipse is being drawn with setRect(const QRectF&). Additionally, you can define whether only a segment of the ellipse should be drawn by calling setStartAngle(int) and setSpanAngle(int). The arguments of both functions are in sixteenths of a degree. |
QGraphicsPolygonItem |
Draws a polygon. You can define the polygon with setPolygon(const QPolygonF&). |
QGraphicsPathItem |
Draws a path, that is, a set of various geometric primitives. You can define the path with setPath(const QPainterPath&). |
QGraphicsSimpleTextItem |
Draws plain text. You can define the text with setText(const QString&) and the font with setFont(const QFont&). This item doesn't support rich formatting. |
QGraphicsTextItem |
Draws formatted text. Unlike QGraphicsSimpleTextItem, this item can display HTML stored in a QTextDocument object. You can set HTML with setHtml(const QString&) and the document with setDocument(QTextDocument*). QGraphicsTextItem can even interact with the displayed text so that text editing or URL opening is possible. |
QGraphicsPixmapItem |
Draws a pixmap (a raster image). You can define the pixmap with setPixmap(const QPixmap&). It's possible to load pixmaps from local files or resources, similar to icons (refer to Chapter 3, Qt GUI Programming, for more information about resources). |
QGraphicsProxyWidget |
Draws an arbitrary QWidget and allows you to interact with it. You can set the widget with setWidget(QWidget*). |
As we already saw, you can usually pass the content of the item to the constructor instead of calling a setter method such as setRect(). However, keep in mind that compact code may be harder to maintain than code that sets all the variables through setter methods.
For most items, you can also define which pen and which brush should be used. The pen is set with setPen() and the brush with setBrush() (we've already used it for the child circles in the previous example). These two functions, however, do not exist for QGraphicsTextItem. To define the appearance of a QGraphicsTextItem item, you have to use setDefaultTextColor() or HTML tags supported by Qt. QGraphicsPixmapItem has no similar methods, as the concepts of pen and brush cannot be applied to pixmaps.
It is generally easier to use standard items than to implement them from scratch. Whenever you will use Graphics View, ask yourself these questions: Which standard items are suited for my specific needs? Am I re-inventing the wheel over and over again? However, from time to time, you need to create custom graphics items, and we'll cover this topic later in this chapter.