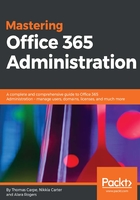
Client-side object model
In your travels, you may come across PowerShell examples that leverage client-side object model (CSOM) to complete tasks in SharePoint Online. CSOM has the benefit of providing granular access to the SharePoint API. It has the shortcoming of requiring the programmer to understand not only the complexity of SharePoint's server-side API but also the nuances of asynchronous communication models used primarily for writing web services.
Nevertheless, there will be many times when CSOM is your only option for automating tasks in SharePoint Online. It's important to understand the basic pattern to connect to a SharePoint site and perform actions using this powerful framework.
You can leverage CSOM by copying the required DLLs of the SharePoint client libraries, using NuGet package manager, or by downloading them directly. Here, we'll use the download, but using NuGet with Visual Studio is the recommended way to keep things current. Download CSOM for SharePoint Online from https://www.microsoft.com/en-us/download/details.aspx?id=42038.
Here's how to connect to a SharePoint web service using SPContext.
First, we need to import the types defined in the CSOM assemblies so we can use them in PowerShell. Note that your DLLs may be stored in a different location depending on how you got them:
$DllPath = "c:\Program Files\Common Files\microsoft shared\Web Server Extensions\15\ISAPI"
# Change $DllPath to wherever you are keeping your assemblies
Add-Type -Path "$DllPath\Microsoft.SharePoint.Client.dll"
Add-Type -Path "$DllPath\Microsoft.SharePoint.Client.Runtime.dll"
# You might need additional references if you need publishing, search, user profiles, etc.
# Add-Type -Path "$DllPath\Microsoft.SharePoint.Client.Taxonomy.dll"
Now, let's set a few variables. To connect to SharePoint, we need the URL for a SharePoint web service and a username/password to connect with:
# Get variables needed to connect to SharePoint website
$Credentials = Get-Credential
$TenantName = "my365tenant"
# Change $TenantName to your Office 365 tenant
# For sub-sites you'll want to modify $WebUrl too
$WebUrl = "https://$TenantName.sharepoint.com"
It's important to understand that CSOM doesn't connect to all of SharePoint at once. Connections are given some content, which is a combination of credentials and a URL that points to a single SharePoint web. This web may be the root of a site collection (sometimes called a site) or it may be a subweb. If you want to connect to a different SharePoint subweb, you'll most likely need a separate ClientContext.
Here's the code to create the context object:
# Convert Credentials to SPO Credentials
$SpoCredentials = New-Object Microsoft.SharePoint.Client.SharePointOnlineCredentials($Credentials.UserName,$Credentials.Password)
$Context = New-Object Microsoft.SharePoint.Client.ClientContext($WebUrl)
$Context.Credentials = $SpoCredentials
$Web = $Context.Web
Now that we're (theoretically) connected, we can get the web title and display it. Before proceeding, we check that $Context has a valid value, indicating the connection was successful:
If (!$Context.ServerObjectIsNull.Value) {
# How to read a client object's properties in CSOM
$Context.Load($Web)
$Context.ExecuteQuery()
$Title = $Web.Title
Write-Host -ForegroundColor Green "Connected to SharePoint Online"
Write-Host "Web url: '$WebUrl'"
Write-Host "Title: '$Title'"
# Now you can write your own code to do CSOM calls against the web context
}
In CSOM, you must first load an object, its properties, or a query. You may do this more than once for different objects or properties. Next, you run that query using the ExecuteQuery function. This pattern reflects the disconnected and asynchronous nature of CSOM, but it does add a great deal of complexity compared to traditional SharePoint's server-side API and PowerShell commands.
Failure to load objects or properties will result in properties with empty values or errors, such as: The collection has not been initialized. It has not been requested or the request has not been executed. It may need to be explicitly requested.
Here's our script in action:

This is a very rudimentary example. To read properties from CSOM and call commands on the server efficiently, you're likely to need a great deal of additional code. See the Other tools and frameworks section for some tips on how to accelerate your efforts.