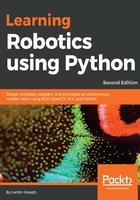
Hello_world_publisher.py
The hello_world_publisher.py node basically publishes a greeting message called hello world to a topic called /hello_pub. The greeting message is published to the topic at a rate of 10 Hz.
Here is a diagram that shows how the interaction between the two ROS nodes works:

Communication between the publisher and subscriber node
The full code of this book is available at https://github.com/qboticslabs/learning_robotics_2nd_ed.
The step-by-step explanation of how this code works is as follows:
- We need to import rospy if we are writing a ROS Python node. It contains Python APIs to interact with ROS topics, services, and so on.
- To send the hello world message, we have to import a String message data type from the std_msgs package. The std_msgs package has the message definition for standard data types. We can import using the following lines of code:
#!/usr/bin/env python import rospy from std_msgs.msg import String
- The following line of code creates a publisher object to a topic called hello_pub. The message type is String and the queue_size value is 10. If the subscriber is not fast enough to receive the data, we can use the queue_size option to buffer it:
def talker(): pub = rospy.Publisher('hello_pub', String, queue_size=10)
- The following line of code initializes a ROS node. It will also assign a name to the node. If two nodes are running with the same node name, one will shut down. If we want to run both, use anonymous=True flag as shown in the following code:
rospy.init_node('hello_world_publisher', anonymous=True)
- The following line creates a rate object called r. Using a sleep() method in the Rate object, we can update the loop at the desired rate. Here, we are giving the rate the value of 10:
r = rospy.Rate(10) # 10hz
- The following loop will check whether rospy constructs the rospy.is_shutdown() flag. Then, it executes the loop. If we click on Ctrl + C, this loop will exit.
Inside the loop, a hello world message is printed on the Terminal and published on the hello_pub topic with a rate of 10 Hz:
while not rospy.is_shutdown(): str = "hello world %s"%rospy.get_time() rospy.loginfo(str) pub.publish(str) r.sleep()
- The following code has Python __main__ check and calls the talker() function. The code will keep on executing the talker(), and when Ctrl + C is pressed the node will get shut down:
if __name__ == '__main__': try: talker() except rospy.ROSInterruptException: pass
After publishing the topic, we will see how to subscribe to it. The following section covers the code needed to subscribe to the hello_pub topic.