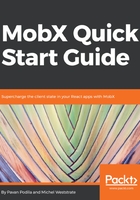
上QQ阅读APP看书,第一时间看更新
Observable arrays
Using observable.array() is very similar to using an observable(). You pass an array as initial value or start with an empty array. In the following code example, we are starting with an empty array:
const items = observable.array(); // Start with empty array
console.log(items.length); // Prints: 0
items.push({
name: 'hats', quantity: 40,
});
// Add one in the front
items.unshift({ name: 'Ribbons', quantity: 2 });
// Add at the back
items.push({ name: 'balloons', quantity: 1 });
console.log(items.length); // Prints: 3
Do note that the observable array is not a real JavaScript array, even though it has the same API as a JS Array. When you are passing this array to other libraries or APIs, you can convert it into a JS Array by calling toJS(), as shown here:
import { observable, toJS } from 'mobx';
const items = observable.array();
/* Add/remove items*/
const plainArray = toJS(items);
console.log(plainArray);
MobX will apply deep observability to observable arrays, which means it will track additions and removals of items from the array and also track property changes happening to each item in the array.