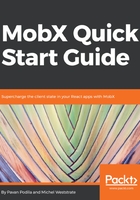
Redux in a nutshell
The data flow triad that we saw earlier is also applicable to Redux in its entirety. It's in the state update mechanism that Redux adds its own twist. This can be seen in the following figure:

When the UI fires the action, it is dispatched on the store. Inside the store, the action first passes through one or more middleware, where it can get acted upon and swallowed without further propagation. If the action passes through the middleware, it is sent to one or more reducers, where it can be processed to produce a new state of the store.
This new state of the store is notified to all of the subscribers, with the UI being one of them. If the state is different from the previous value that the UI has, the UI is re-rendered and brought in sync with the new state.
There are few things here that are worth highlighting:
- From the point where the action enters the store, until the new state is computed, the entire process is synchronous.
- Reducers are pure functions that take in the action and the previous state and produce the new state. Since they are pure functions, you cannot put side effects, such as a network call, inside a reducer.
- Middleware is the only place where side effects can be performed, eventually resulting in an action being dispatched on the store.
If you are using Redux with React, which is the most likely combination, there is a utility library called react-redux, which can glue the store with React components. It does this through a function called connect(), which binds the store with the passed in React component. Inside connect(), the React component subscribes to the store for state-change notifications. Binding to the store via connect() means that every state change is notified to every component. This requires adding additional abstractions, such as a state-selector (using mapStateToProps) or implementing shouldComponentUpdate() to receive only the relevant state updates:
connect(mapStateToProps, mapDispatchToProps, mergeProps, options)(Component)
We are deliberately skipping over a few other details that are required for a complete React-Redux setup, but the essentials are in place for a deeper comparison of Redux with MobX.