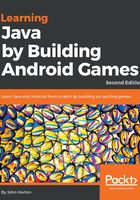
Introduction to Java methods
Java methods are a way of organizing and compartmentalizing our code. They are quite a deep topic and a full understanding requires knowledge of other Java topics. By the end of the book you will be a method Ninja but for now, a basic introduction will be useful.
Methods have names to identify them from other methods and to help the programmer identify what they do. The methods in the Sub' Hunter game will have names like draw
, takeShot
, newGame,
and printDebuggingText
as well as a few more.
Code with a specific purpose can be wrapped inside a method, perhaps like this:
void draw(){ // Handle all the drawing here }
The above method called draw
could hold all the lines of code that does the drawing for our game. When we set out a method with its code it is called the method definition. The curious looking prefixed void
keyword and the postfixes ()
will be explained in Chapter 4, Structuring Code with Java Methods but for now, you just need to know that all the code inside the draw
method will be executed when another part of the code wants it to be executed.
When we want to initiate a method from another part of the code, we say that we call the method. And we would call the draw
method with this code:
draw();
Note the following, especially the last point, it is very important:
- Methods can call other methods
- We can call methods as many times as we want
- The order in which the method definitions appear in the code file doesn't matter. If the definition exists, it can be called from the code in that file.
- When the called method has completed execution the program execution returns to the line after the method call.
So, in our example program flow would look like this.
… // Going to go to the draw method now draw(); // Back from the draw method // Any more code here executes next …
Note
In Chapter 8, Object-Oriented Programming we will also see how we can call methods in one file from another file.
By coding the logic of the Sub' Hunter game into methods and calling the appropriate methods from other appropriate methods we can implement the flow of actions indicated in the flowchart.