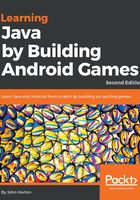
Abstract classes
An abstract class is a class that cannot be instantiated, cannot be made into an object. We saw this in the inheritance mini-app.
Note
So, it's a blueprint that will never be used then? But that's like paying an architect to design your home and then never building it? You might be saying to yourself, "I kind of got the idea of an abstract method but abstract classes are just silly."
If we or the designer of a class wants to force us to inherit before we use their class, they can declare a class abstract. Then, we cannot make an object from it; therefore, we must extend it first and make an object from the subclass.
We can also declare a method abstract
and then that method must be overridden in any class that extends the class with the abstract method.
Let's look at an example, it will help. We make a class abstract by declaring it with the abstract
keyword like this.
abstract class someClass{ /* All methods and variables here. As usual- Just don't try and make an object out of me! */ }
Yes, but why, you might fairly ask?
Sometimes we want a class that can be used as a polymorphic type, but we need to guarantee it can never be used as an object. For example, Animal doesn't really make sense on its own.
We don't talk about animals we talk about types of animals. We don't say, "Ooh, look at that lovely fluffy, white animal". Or, "yesterday we went to the pet shop and got an animal and an animal bed". It's just too, well, abstract.
So, an abstract class is kind of like a template to be used by any class that extends
it (inherits from it).
We might want a Worker
class and extend to make, Miner
, Steelworker
, OfficeWorker
, and of course Programmer
. But what exactly does a plain Worker
do? Why would we ever want to instantiate one?
The answer is we wouldn't want to instantiate one; but we might want to use it as a polymorphic type, so we can pass multiple worker subclasses between methods and have data structures that can hold all types of Worker
.
This is the point of an abstract class and when a class has even one abstract method it must be declared abstract itself. And all abstract methods must be overridden by any class which extends it. This means that the abstract class can provide some of the common functionality that would be available in all its subclasses.
For example, the Worker
class might have the height
, weight
, and age
member variables. It might have the getPayCheck
method which is not abstract and is the same in all the subclasses, but a doWork
method which is abstract and must be overridden, because all the different types of worker doWork
very differently.
Which leads us neatly to another area of polymorphism that is going to make life easier for us throughout this book.