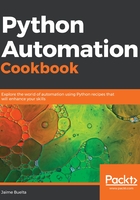
上QQ阅读APP看书,第一时间看更新
There's more...
Please check out the full documentation of configparse in the official Python documentation: https://docs.python.org/3/library/configparser.html.
In most cases, this configuration parser should be good enough, but if more power is needed, you can use YAML files as configuration files. YAML files (https://learn.getgrav.org/advanced/yaml) are very common as configuration files, and are better structured and can be parsed directly, taking into account data types.
- Add PyYAML to the requirements.txt file and install it:
PyYAML==3.12
- Create the prepare_task_yaml.py file:
import yaml
import argparse
import sys
def main(number, other_number, output):
result = number * other_number
print(f'The result is {result}', file=output)
if __name__ == '__main__':
parser = argparse.ArgumentParser()
parser.add_argument('-n1', type=int, help='A number', default=1)
parser.add_argument('-n2', type=int, help='Another number', default=1)
parser.add_argument('-c', dest='config', type=argparse.FileType('r'),
help='config file in YAML format',
default=None)
parser.add_argument('-o', dest='output', type=argparse.FileType('w'),
help='output file',
default=sys.stdout)
args = parser.parse_args()
if args.config:
config = yaml.load(args.config)
# No need to transform values
args.n1 = config['ARGUMENTS']['n1']
args.n2 = config['ARGUMENTS']['n2']
main(args.n1, args.n2, args.output)
- Define the config file config.yaml, available in GitHub https://github.com/PacktPublishing/Python-Automation-Cookbook/blob/master/Chapter02/config.yaml :
ARGUMENTS:
n1: 7
n2: 4
- Then, run the following:
$ python3 prepare_task_yaml.py -c config.yaml
The result is 28
There's also the possibility of setting a default config file, as well as a default output file. This can be handy to create a pure task that requires no input parameters.
As a general rule, try to avoid creating too many input and configuration parameters if the task has a very specific objective in mind. Try to limit the input parameters to different executions of the task. A parameter that never changes is probably fine being defined as a constant. A high number of parameters will make config files or command-line arguments complicated and will create more maintenance in the long run. On the other hand, if your objective is to create a very flexible tool to be used in very different situations, then creating more parameters is probably a good idea. Try to find your own proper balance!