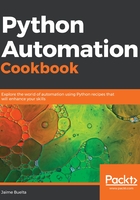
上QQ阅读APP看书,第一时间看更新
How to do it...
- Prepare the following task and save it as prepare_task_step1.py:
import argparse
def main(number, other_number):
result = number * other_number
print(f'The result is {result}')
if __name__ == '__main__':
parser = argparse.ArgumentParser()
parser.add_argument('-n1', type=int, help='A number', default=1)
parser.add_argument('-n2', type=int, help='Another number', default=1)
args = parser.parse_args()
main(args.n1, args.n2)
- Update the file to define a config file that contains both arguments, and save it as prepare_task_step2.py. Note that defining a config file overwrites any command-line parameters:
import argparse
import configparser
def main(number, other_number):
result = number * other_number
print(f'The result is {result}')
if __name__ == '__main__':
parser = argparse.ArgumentParser()
parser.add_argument('-n1', type=int, help='A number', default=1)
parser.add_argument('-n2', type=int, help='Another number', default=1)
parser.add_argument('--config', '-c', type=argparse.FileType('r'),
help='config file')
args = parser.parse_args()
if args.config:
config = configparser.ConfigParser()
config.read_file(args.config)
# Transforming values into integers
args.n1 = int(config['DEFAULT']['n1'])
args.n2 = int(config['DEFAULT']['n2'])
main(args.n1, args.n2)
- Create the config file config.ini:
[ARGUMENTS]
n1=5
n2=7
- Run the command with the config file. Note that the config file overwrites the command-line parameters, as described in step 2:
$ python3 prepare_task_step2.py -c config.ini
The result is 35
$ python3 prepare_task_step2.py -c config.ini -n1 2 -n2 3
The result is 35
- Add a parameter to store the result in a file, and save it as prepare_task_step5.py:
import argparse
import sys
import configparser
def main(number, other_number, output):
result = number * other_number
print(f'The result is {result}', file=output)
if __name__ == '__main__':
parser = argparse.ArgumentParser()
parser.add_argument('-n1', type=int, help='A number', default=1)
parser.add_argument('-n2', type=int, help='Another number', default=1)
parser.add_argument('--config', '-c', type=argparse.FileType('r'),
help='config file')
parser.add_argument('-o', dest='output', type=argparse.FileType('w'),
help='output file',
default=sys.stdout)
args = parser.parse_args()
if args.config:
config = configparser.ConfigParser()
config.read_file(args.config)
# Transforming values into integers
args.n1 = int(config['DEFAULT']['n1'])
args.n2 = int(config['DEFAULT']['n2'])
main(args.n1, args.n2, args.output)
- Run the result to check that it's sending the output to the defined file. Note that there's no output outside the result files:
$ python3 prepare_task_step5.py -n1 3 -n2 5 -o result.txt
$ cat result.txt
The result is 15
$ python3 prepare_task_step5.py -c config.ini -o result2.txt
$ cat result2.txt
The result is 35