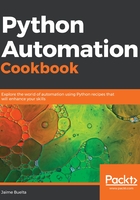
上QQ阅读APP看书,第一时间看更新
How to do it...
- Import the parse function:
>>> from parse import parse
- Define the log to parse, in the same format as in the Extracting data from structured strings recipe:
>>> LOG = '[2018-05-06T12:58:00.714611] - SALE - PRODUCT: 1345 - PRICE: $09.99'
- Analyze it and describe it as you'll do when trying to print it, like this:
>>> FORMAT = '[{date}] - SALE - PRODUCT: {product} - PRICE: ${price}'
- Run parse and check the results:
>>> result = parse(FORMAT, LOG)
>>> result
<Result () {'date': '2018-05-06T12:58:00.714611', 'product': '1345', 'price': '09.99'}>
>>> result['date']
'2018-05-06T12:58:00.714611'
>>> result['product']
'1345'
>>> result['price']
'09.99'
- Note the results are all strings. Define the types to be parsed:
>>> FORMAT = '[{date:ti}] - SALE - PRODUCT: {product:d} - PRICE: ${price:05.2f}'
- Parse once again:
>>>result = parse(FORMAT, LOG)
>>> result
<Result () {'date': datetime.datetime(2018, 5, 6, 12, 58, 0, 714611), 'product': 1345, 'price': 9.99}>
>>> result['date']
datetime.datetime(2018, 5, 6, 12, 58, 0, 714611)
>>> result['product']
1345
>>> result['price']
9.99
- Define a custom type for the price to avoid issues with the float type:
>>> from decimal import Decimal
>>> def price(string):
... return Decimal(string)
...
>>> FORMAT = '[{date:ti}] - SALE - PRODUCT: {product:d} - PRICE: ${price:price}'
>>> parse(FORMAT, LOG, {'price': price})
<Result () {'date': datetime.datetime(2018, 5, 6, 12, 58, 0, 714611), 'product': 1345, 'price': Decimal('9.99')}>