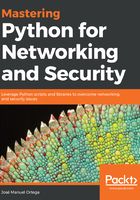
Implementing a Server with socket.io
The Socket.IO server is available in the official python repository and can be installed via pip: pip install python-socketio.
The full documentation is available at https://python-socketio.readthedocs.io/en/latest/.
The following is an example that works in python 3.5 where we implement a Socket.IO server using the aiohttp framework for asyncio:
from aiohttp import web
import socketio
socket_io = socketio.AsyncServer()
app = web.Application()
socket_io.attach(app)
async def index(request):
return web.Response(text='Hello world from socketio' content_type='text/html')
# You will receive the new messages and send them by socket
@socket_io.on('message')
def print_message(sid, message):
print("Socket ID: " , sid)
print(message)
app.router.add_get('/', index)
if __name__ == '__main__':
web.run_app(app)
In the previous code, we implemented a server based on socket.io that uses the aiohttp module. As you can see in the code, we define two methods, the index () method, which will return a response message upon receiving a request on the "/" root endpoint, and a print_message () method that contains the @socketio.on (' message ') annotation. This annotation causes the function to listen for message-type events, and when these events occur, it will act on those events.