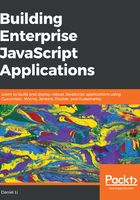
Migrating our API to Express
There are two ways to install Express: directly in the code itself or through the express-generator application generator tool. The express-generator tool installs the express CLI, which we can use to generate an application skeleton from. However, we won't be using that because it's mainly meant for client-facing applications, while we are just trying to build a server-side API at the moment. Instead, we'll add the express package directly into our code.
First, add the package into our project:
$ yarn add express
Now open up your src/index.js file, and replace our import of the http module with the express package. Also replace the current http.createServer and server.listen calls with express and app.listen. What was previously this:
...
import http from 'http';
...
const server = http.createServer(requestHandler);
server.listen(8080);
Would now be this:
...
import express from 'express';
...
const app = express();
app.listen(process.env.SERVER_PORT);
To help us know when the server has successfully initialized, we should add a callback function to app.listen, which will log a message onto the console:
app.listen(process.env.SERVER_PORT, () => {
// eslint-disable-next-line no-console
console.log(`Hobnob API server listening on port ${process.env.SERVER_PORT}!`);
});
We needed to disable ESLint for our console.log line because Airbnb's style guide enforces the no-console rule. // eslint-disable-next-line is a special type of comment recognized by ESLint, and will cause it to disable the specified rules for the next line. There is also the // eslint-disable-line comment if you want to disable the same line as the comment.