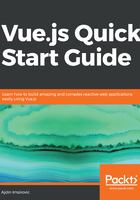
Computed properties and watchers
Computed properties are used to avoid complex logic adding bloat to your views. In other words, computed properties are useful to hide the complexity from our HTML and thus keep our HTML understandable, easy to use, and declarative. Put differently, when we need to compute some values from the data option, we can do that with the help of computed properties.
The full code for the following example can be seen at https://codepen.io/AjdinImsirovic/pen/WyXEOz:
<!-- HTML -->
<div id="example">
<p>User name: "{{ message }}"</p>
<p>Message prefixed with a title: "{{ prefixedMessage }}"</p>
</div>
// JS
var example = new Vue({
el: '#example',
data: {
userName: 'John Doe',
title: ''
},
computed: {
// a computed getter
prefixedMessage: function () {
// `this` points to the Vue instance's data option
return this.title + " " + this.userName
}
}
})
Watchers are not as frequently used as computed properties are. In other words, the watch option is to be used less frequently than the computed properties option. Watchers are commonly used for asynchronous or otherwise costly operations with changing data.
Watchers have to do with reactive programming; they allow us to observe a sequence of events through time and react to changes as they happen on a certain data property.
We will cover the subject of computed properties and watchers in later chapters. For now, it is sufficient to know that they exist in Vue and that they are widely used.