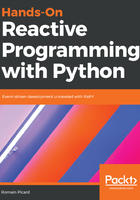
The repeat operator
The repeat operator allows for repeating the emission of an item or a sequence. The marble diagram of the repeat operator is shown in the following figure:
This operator has two prototypes, as follows:
Observable.repeat(value=None, repeat_count=None, scheduler=None)
Observable.repeat(self, repeat_count=None)
The first implementation is a static class method. It can be used to create an observable that repeats value items for repeat_count times. If the value is not set, items with a None value are emitted. The second implementation is a method that repeats its source observable for repeat_count times. In both methods, the repeat_count argument is optional. When repeat_count is not set, the repetition is infinite. If the repeat_count is less than one, then no items are emitted.
The first variant of the implementation can be used in the following way:
ones = Observable.repeat(1, 5)
ones.subscribe(
on_next=lambda i: print("item: {}".format(i)),
on_error=lambda e: print("error: {}".format(e)),
on_completed=lambda: print("completed")
)
The preceding code will provide the following output:
The second variant is used in a chain of operators, like in the following simple example:
numbers = Observable.from_([1,2,3])
numbers.repeat(3).subscribe(
on_next=lambda i: print("item: {}".format(i)),
on_error=lambda e: print("error: {}".format(e)),
on_completed=lambda: print("completed")
)
First, the numbers observable is created. Upon subscription, it emits the sequence 1, 2, 3. This sequence is then repeated three times. This provides the following result: