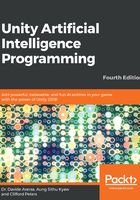
上QQ阅读APP看书,第一时间看更新
The Patrol state
While our tank is in the Patrol state, we check whether it has reached the destination point. If so, it finds the next destination point to follow. The FindNextPoint method basically chooses the next random destination point among the waypoints defined. If it's still on the way to the current destination point, it'll check the distance to the player's tank. If the player's tank is in range (which we choose to be 300 here), then it switches to the Chase state. The rest of the code just rotates the tank and moves forward:
protected void UpdatePatrolState() { //Find another random patrol point if the current //point is reached if (Vector3.Distance(transform.position, destPos) <= 100.0f) { print("Reached to the destination point\n"+ "calculating the next point"); FindNextPoint(); } //Check the distance with player tank //When the distance is near, transition to chase state else if (Vector3.Distance(transform.position, playerTransform.position) <= 300.0f) { print("Switch to Chase Position"); curState = FSMState.Chase; } //Rotate to the target point Quaternion targetRotation = Quaternion.LookRotation(destPos - transform.position); transform.rotation = Quaternion.Slerp(transform.rotation, targetRotation, Time.deltaTime * curRotSpeed); //Go Forward transform.Translate(Vector3.forward * Time.deltaTime * curSpeed); } protected void FindNextPoint() { print("Finding next point"); int rndIndex = Random.Range(0, pointList.Length); float rndRadius = 10.0f; Vector3 rndPosition = Vector3.zero; destPos = pointList[rndIndex].transform.position + rndPosition; //Check Range to decide the random point //as the same as before if (IsInCurrentRange(destPos)) { rndPosition = new Vector3(Random.Range(-rndRadius, rndRadius), 0.0f, Random.Range(-rndRadius, rndRadius)); destPos = pointList[rndIndex].transform.position + rndPosition; } } protected bool IsInCurrentRange(Vector3 pos) { float xPos = Mathf.Abs(pos.x - transform.position.x); float zPos = Mathf.Abs(pos.z - transform.position.z); if (xPos <= 50 && zPos <= 50) return true; return false; }