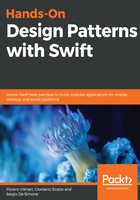
Dangling references
Now that we've seen the powerful weak and unowned modifiers and have regained control over our memory management, we are thrilled to use it everywhere. That is, until we see a crash in the form of the following:
Fatal error: Attempted to read an unowned reference but object 0x10132c0f0 was already deallocated.
This is the same issue as the, which the dangling pointer one that we saw earlier in this chapter.
Let's reuse the example, as follows:
func getCard() -> Card {
let batman = Person(name: "Batman")
let card = Card(batman)
batman.cards.append(card)
return card
}
let card = getCard()
print("\(card.owner.name)")
In this code, we explicitly return a card, but the owner of this card is not in the memory anymore, as it was properly deallocated after we exited the scope. Let's break it down, line by line, and try to get an idea of the counts, as shown in the following table:

With this in mind, we can now see why the program crashes, as there's nothing left to retain the owner of the card.
This can be seen as unsafe, but it should not be. This example, while valid, is a perfect example where the relationship is unowned because it can't be anything else. weak would have forced us to write the owner as an optional, which is incorrect, and using a strong relationship ultimately leads to cycles and leaks being retained.
To overcome this issue, we have to re-evaluate our code and ensure that we never return a card disassociated from its owner. The unowned references preserve the semantics of your program, while ensuring that the memory management is sane.